勉強になります。
ありがとうございます。
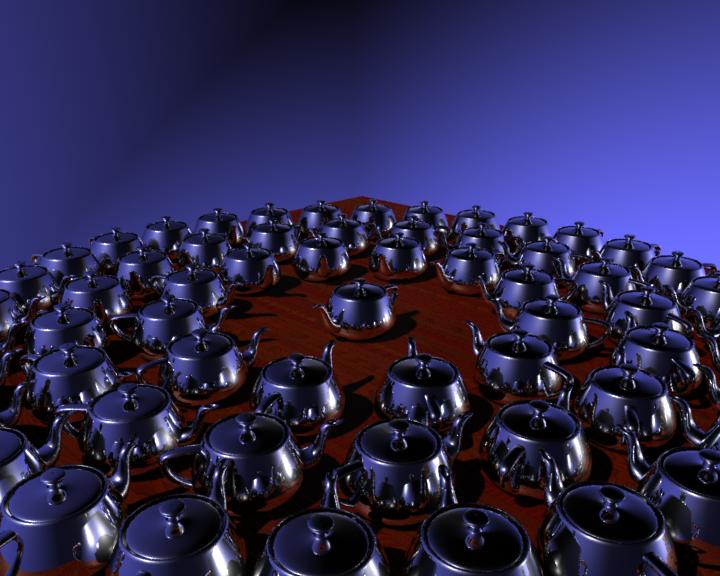
#!/usr/bin/python
# set PYTHONPATH=C:\Python25;C:\Python25\Scripts;%RMANTREE%\bin
import sys
sys.path.append("D:\lighting\PythonClasses")
import math
import getpass
import time,random
# import the python renderman library
import prman
from vector import Vector
from Camera import *
def Scene(ri) :
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
random.seed(25)
face=[-0.1,-1,-10, 0.1,-1,-10,-0.1,-1,10, 0.1,-1,10]
plank=-10.0
while (plank <=10.0) :
ri.TransformBegin()
ri.Color([random.uniform(0.35,0.4),random.uniform(0.1,0.025),0])
c0=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
c1=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
ri.Surface("wood",{"Ks":[0.1],"point c0":c0,"point c1":c1,"float grain":random.randint(2,20)})
ri.Translate(plank,0,0)
ri.Patch("bilinear",{'P':face})
ri.TransformEnd()
plank=plank+0.206
ri.AttributeEnd()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
face=[-10,-1,-10, 10,-1,-10,-10,-1,10, 10,-1,10]
ri.TransformBegin()
ri.Color([0.1,0.1,0.1])
ri.Translate(0,-0.5,0.8)
ri.Patch("bilinear",{'P':face})
ri.TransformEnd()
ri.AttributeEnd()
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.9,0.9,0.9])
ri.Translate( 0,-1.0,0)
ri.Rotate(-90,1,0,0)
ri.Rotate(0,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface( "shinymetal", {"string texturename": ["raytrace"]})
#ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
for i in range(0,361,12):
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.9,0.9,0.9])
ri.Rotate(i,0,1,0)
ri.Translate( 0,-1.0,8)
ri.Rotate(-90,1,0,0)
ri.Rotate(90,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface( "shinymetal", {"string texturename": ["raytrace"]})
#ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
for i in range(0,361,20):
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.9,0.9,0.9])
ri.Rotate(i,0,1,0)
ri.Translate( 0,-1.0,6)
ri.Rotate(-90,1,0,0)
ri.Rotate(30,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface( "shinymetal", {"string texturename": ["raytrace"]})
#ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
for i in range(0,361,30):
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.9,0.9,0.9])
ri.Rotate(i,0,1,0)
ri.Translate( 0,-1.0,4)
ri.Rotate(-90,1,0,0)
ri.Rotate(80,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface( "shinymetal", {"string texturename": ["raytrace"]})
#ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
ri = prman.Ri() # create an instance of the RenderMan interface
#cam=Camera(Vector(4,0.2,4,1),Vector(0,0,0,1),Vector(0,1,0,0))
cam=Camera(Vector(10,5,-10,1),Vector(0,0,0,1),Vector(0,1,0,0))
cam.fov=45
#filename = "Camera.rib"
filename = "__render"
ri.Begin(filename)
#ri.Begin(ri.RENDER)
ri.Declare("Light1" ,"string")
ri.Declare("Light2" ,"string")
ri.Declare("Light3" ,"string")
# now we add the display element using the usual elements
# FILENAME DISPLAY Type Output format
ri.Display("Camera.exr", "framebuffer", "rgba")
#ri.Display("Camera02.png", "file", "rgb")
cam.Format(ri)
# now we start our world
ri.WorldBegin()
ri.LightSource("shadowdistant",{ri.HANDLEID:"Light1", "from": [ -10, 10, -10], "to": [ 0, 0, 0], "intensity":[2.0], "string shadowname": ["raytrace"]})
#ri.LightSource( "pointlight", {ri.HANDLEID:"Light1", "point from":[-2,2,4], "float intensity": [50]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light2", "point to":[-1,-0.03,0], "float intensity": [1]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light3", "point to":[0,-0.5,-1], "float intensity": [0.2]})
ri.Illuminate("Light1",1)
ri.Illuminate("Light2",1)
ri.Illuminate("Light3",1)
cam.Place(ri)
ri.AttributeBegin()
size=20.0
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [0]})
ri.TransformBegin()
ri.Color(( .2773, .2855, .6679))
ri.Surface( "matte")
ri.Sphere(size, -size, size,360)
ri.TransformEnd()
ri.AttributeEnd()
ri.TransformBegin()
Scene(ri)
ri.TransformEnd()
# end our world
ri.WorldEnd()
# and finally end the rib file
ri.End()
- -
- -