1/2 >>
Pythonを使ってRenderManを操る その1
とりあえず、サンプルを参考にカメラをテストしました。
イギリスのbournemouth universityを参考にしました。ありがとうございます。Thank you.
#!/usr/bin/python
# set PYTHONPATH=C:\Python25;C:\Python25\Scripts;%RMANTREE%\bin
import sys
sys.path.append("D:\lighting\PythonClasses")
import math
import getpass
import time,random
# import the python renderman library
import prman
from vector import Vector
from Camera import *
def Scene(ri) :
ri.AttributeBegin()
random.seed(25)
face=[-0.1,-1,-3, 0.1,-1,-3,-0.1,-1,3, 0.1,-1,3]
plank=-5.0
while (plank <=5.0) :
ri.TransformBegin()
ri.Color([random.uniform(0.35,0.4),random.uniform(0.1,0.025),0])
c0=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
c1=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
ri.Surface("wood",{"Ks":[0.1],"point c0":c0,"point c1":c1,"float grain":random.randint(2,20)})
ri.Translate(plank,0,0)
ri.Patch("bilinear",{'P':face})
ri.TransformEnd()
plank=plank+0.206
ri.AttributeEnd()
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.2,0.4,0.75])
ri.Translate( 0,-1.0,0)
ri.Rotate(-90,1,0,0)
ri.Rotate(36,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
ri = prman.Ri() # create an instance of the RenderMan interface
#cam=Camera(Vector(4,0.2,4,1),Vector(0,0,0,1),Vector(0,1,0,0))
cam=Camera(Vector(0,2,4,1),Vector(0,0,0,1),Vector(0,1,0,0))
cam.fov=40
#filename = "Camera.rib"
filename = "__render"
ri.Begin(filename)
#ri.Begin(ri.RENDER)
ri.Declare("Light1" ,"string")
ri.Declare("Light2" ,"string")
ri.Declare("Light3" ,"string")
# now we add the display element using the usual elements
# FILENAME DISPLAY Type Output format
#ri.Display("Camera.exr", "framebuffer", "rgba")
ri.Display("Camera.png", "file", "rgb")
cam.Format(ri)
# now we start our world
ri.WorldBegin()
ri.LightSource("shadowdistant",{ri.HANDLEID:"Light1", "from": [ -10, 10, -10], "to": [ 0, 0, 0], "intensity":[2.0], "string shadowname": ["raytrace"]})
#ri.LightSource( "pointlight", {ri.HANDLEID:"Light1", "point from":[-2,2,4], "float intensity": [50]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light2", "point to":[-1,-0.03,0], "float intensity": [1]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light3", "point to":[0,-0.5,-1], "float intensity": [0.2]})
ri.Illuminate("Light1",1)
ri.Illuminate("Light2",1)
ri.Illuminate("Light3",1)
cam.Place(ri)
ri.TransformBegin()
Scene(ri)
ri.TransformEnd()
# end our world
ri.WorldEnd()
# and finally end the rib file
ri.End()
Pythonを使ってRenderManを操る その2
イギリスのbournemouth universityを参考にしました。ありがとうございます。Thank you.
#!/usr/bin/python
# set PYTHONPATH=C:\Python25;C:\Python25\Scripts;%RMANTREE%\bin
import sys
sys.path.append("D:\lighting\PythonClasses")
import math
import getpass
import time,random
# import the python renderman library
import prman
from vector import Vector
from Camera import *
def Scene(ri) :
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
random.seed(25)
face=[-0.1,-1,-3, 0.1,-1,-3,-0.1,-1,3, 0.1,-1,3]
plank=-5.0
while (plank <=5.0) :
ri.TransformBegin()
ri.Color([random.uniform(0.35,0.4),random.uniform(0.1,0.025),0])
c0=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
c1=[random.uniform(-10,10),random.uniform(-10,10),random.uniform(-10,10)]
ri.Surface("wood",{"Ks":[0.1],"point c0":c0,"point c1":c1,"float grain":random.randint(2,20)})
ri.Translate(plank,0,0)
ri.Patch("bilinear",{'P':face})
ri.TransformEnd()
plank=plank+0.206
ri.AttributeEnd()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
face=[-5,-1,-5, 5,-1,-5,-5,-1,5, 5,-1,5]
ri.TransformBegin()
ri.Color([0.1,0.1,0.1])
ri.Translate(0,-0.5,0.8)
ri.Patch("bilinear",{'P':face})
ri.TransformEnd()
ri.AttributeEnd()
ri.TransformBegin()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.Color([0.9,0.9,0.9])
ri.Translate( 0,-1.0,0)
ri.Rotate(-90,1,0,0)
ri.Rotate(36,0,0,1)
ri.Scale(0.4,0.4,0.4)
ri.Surface( "shinymetal", {"string texturename": ["raytrace"]})
#ri.Surface("plastic")
ri.Geometry("teapot")
ri.AttributeEnd()
ri.TransformEnd()
ri.AttributeBegin()
size=0.5
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.TransformBegin()
ri.Color(( 1, 0.1, 0))
ri.Surface( "plastic")
ri.Translate( 1.05, -0.5, 1.5)
ri.Rotate( -90, 1, 0, 0)
ri.Sphere(size, -size, size,360)
ri.TransformEnd()
ri.AttributeEnd()
ri.AttributeBegin()
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [1]})
ri.TransformBegin()
ri.Color(( 0.1, 1, 0))
ri.Surface( "plastic")
ri.Translate( -1.45, -0.5, 1.2)
ri.Rotate( -90, 1, 0, 0)
ri.Sphere(size, -size, size,360)
ri.TransformEnd()
ri.AttributeEnd()
ri = prman.Ri() # create an instance of the RenderMan interface
#cam=Camera(Vector(4,0.2,4,1),Vector(0,0,0,1),Vector(0,1,0,0))
cam=Camera(Vector(0,2,4,1),Vector(0,-0.5,0,1),Vector(0,1,0,0))
cam.fov=45
#filename = "Camera.rib"
filename = "__render"
ri.Begin(filename)
#ri.Begin(ri.RENDER)
ri.Declare("Light1" ,"string")
ri.Declare("Light2" ,"string")
ri.Declare("Light3" ,"string")
# now we add the display element using the usual elements
# FILENAME DISPLAY Type Output format
#ri.Display("Camera.exr", "framebuffer", "rgba")
ri.Display("Camera02.png", "file", "rgb")
cam.Format(ri)
# now we start our world
ri.WorldBegin()
ri.LightSource("shadowdistant",{ri.HANDLEID:"Light1", "from": [ -10, 10, -10], "to": [ 0, 0, 0], "intensity":[2.0], "string shadowname": ["raytrace"]})
#ri.LightSource( "pointlight", {ri.HANDLEID:"Light1", "point from":[-2,2,4], "float intensity": [50]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light2", "point to":[-1,-0.03,0], "float intensity": [1]})
ri.LightSource( "distantlight", {ri.HANDLEID:"Light3", "point to":[0,-0.5,-1], "float intensity": [0.2]})
ri.Illuminate("Light1",1)
ri.Illuminate("Light2",1)
ri.Illuminate("Light3",1)
cam.Place(ri)
ri.AttributeBegin()
size=20.0
ri.Attribute( "visibility", {"trace": [1]})
ri.Attribute( "visibility", {"int transmission": [0]})
ri.TransformBegin()
ri.Color(( .2773, .2855, .6679))
ri.Surface( "matte")
ri.Sphere(size, -size, size,360)
ri.TransformEnd()
ri.AttributeEnd()
ri.TransformBegin()
Scene(ri)
ri.TransformEnd()
# end our world
ri.WorldEnd()
# and finally end the rib file
ri.End()
カメラ位置
cam=Camera(Vector(0,0,4,1),Vector(0,-0.5,0,1),Vector(0,1,0,0))
カメラ位置
cam=Camera(Vector(0,2,4,1),Vector(0,-0.5,0,1),Vector(0,1,0,0))
はじめてのFujiyama Renderer その6
カメラ周りの視野変換をRenderManで使うplacecam.cを参考に組んでみた。調整が必要ですが何とか動いています。
以下は、Pov-Rayのシーンサンプルを参考に組んでみました。fovの設定もわかりました。
#!/usr/bin/env python
# 2 teapots and 2 bunnies with 1 sphere light
# Copyright (c) 2011-2013 Hiroshi Tsubokawa
#export PYTHONPATH=$PYTHONPATH:/usr/lib/python2.6/site-packages/:$HOME/Fujiyama-Renderer/bin/
import fujiyama
si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
#Camera
si.NewCamera('cam1', 'PerspectiveCamera')
#si.SetSampleProperty3('cam1', 'translate', 0, 8, 8, 0)
#si.SetSampleProperty3('cam1', 'rotate', -45, 0, 0, 0)
#Calculate python fujicam.py 0 2 -10 0 1 0
si.SetSampleProperty3('cam1', 'rotate',-5.71, 0.00, 0.00,0)
si.SetSampleProperty3('cam1', 'rotate',0.00, 180.00, 0.00,0)
si.SetSampleProperty3('cam1', 'translate', 0.00, 2.00, -10.00,0)
si.SetProperty1('cam1', 'fov', 25)
#Light
si.NewLight('light1', 'SphereLight')
si.SetProperty3('light1', 'translate', -10, 10, -10)
si.SetProperty3('light1', 'scale', .5, .5, .5)
si.SetProperty1('light1', 'intensity', 1)
si.NewLight('light2', 'SphereLight')
si.SetProperty3('light2', 'translate', 10, 10, -10)
si.SetProperty3('light2', 'scale', .5, .5, .5)
si.SetProperty1('light2', 'intensity', 1)
si.SetProperty1('light1', 'sample_count', 16)
#Texture
si.NewTexture('tex1', '../mip/pisa.mip')
#Shader
si.NewShader('dome_shader', 'ConstantShader')
si.AssignTexture('dome_shader', 'texture', 'tex1')
si.NewShader('floor_shader', 'PlasticShader')
si.SetProperty3('floor_shader', 'diffuse', .2, .25, .3)
intensity = 0
si.NewShader('sphere_shader', 'PlasticShader')
si.SetProperty3('sphere_shader', 'diffuse', intensity, intensity, intensity)
si.SetProperty1('sphere_shader', 'ior', 40)
#Mesh
si.NewMesh('dome_mesh', '../mesh/dome.mesh')
si.NewMesh('floor_mesh', '../mesh/floor.mesh')
si.NewMesh('sphere_mesh', '../mesh/sphere.mesh')
#ObjectInstance
si.NewObjectInstance('dome1', 'dome_mesh')
si.SetProperty3('dome1', 'rotate', 0, 0, 0)
si.SetProperty3('dome1', 'scale', -.5, .5, .5)
si.AssignShader('dome1', 'dome_shader')
distance = 1.5
si.NewObjectInstance('floor1', 'floor_mesh')
si.SetProperty3('floor1', 'scale', 5, 5, 5)
si.AssignShader('floor1', 'floor_shader')
si.NewObjectInstance('sphere1', 'sphere_mesh')
si.AssignShader('sphere1', 'sphere_shader')
si.SetProperty3('sphere1', 'translate', 0, 1, 0)
#si.SetProperty3('sphere1', 'scale', .5, .5, .5)
#ObjectGroup
si.NewObjectGroup('group1')
si.AddObjectToGroup('group1', 'sphere1')
si.AssignObjectGroup('sphere1', 'shadow_target', 'group1')
si.AssignObjectGroup('floor1', 'shadow_target', 'group1')
#FrameBuffer
si.NewFrameBuffer('fb1', 'rgba')
#Renderer
si.NewRenderer('ren1')
si.AssignCamera('ren1', 'cam1')
si.AssignFrameBuffer('ren1', 'fb1')
si.SetProperty2('ren1', 'resolution', 640, 480)
#si.SetProperty2('ren1', 'resolution', 160, 120)
si.SetProperty2('ren1', 'pixelsamples', 12, 12)
#Rendering
si.RenderScene('ren1')
#Output
si.SaveFrameBuffer('fb1', '../povsphere01.fb')
#Run commands
si.Run()
#si.Print()
はじめてのFujiyama Renderer その7
domeに張り付けた景色を回転させるシーンを作ってみた。pythonの繰り返しを利用している。10度ずつ回転させて36枚の静止画を生成してみます。
#!/usr/bin/env python
# povsphere02.py
# Copyright (c) 2011-2013 Hiroshi Tsubokawa
#export PYTHONPATH=$PYTHONPATH:/usr/lib/python2.6/site-packages/:$HOME/Fujiyama-Renderer/bin/
import fujiyama
rot=0
for f in range(1,37):
si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
#Camera
si.NewCamera('cam1', 'PerspectiveCamera')
#si.SetSampleProperty3('cam1', 'translate', 0, 8, 8, 0)
#si.SetSampleProperty3('cam1', 'rotate', -45, 0, 0, 0)
#Calculate python fujicam.py 0 2 -10 0 1 0
si.SetSampleProperty3('cam1', 'rotate',-5.71, 0.00, 0.00,0)
si.SetSampleProperty3('cam1', 'rotate',0.00, 180.00, 0.00,0)
si.SetSampleProperty3('cam1', 'translate', 0.00, 2.00, -10.00,0)
si.SetProperty1('cam1', 'fov', 25)
#Light
si.NewLight('light1', 'SphereLight')
si.SetProperty3('light1', 'translate', -10, 10, -10)
si.SetProperty3('light1', 'scale', .5, .5, .5)
si.SetProperty1('light1', 'intensity', 1)
si.NewLight('light2', 'SphereLight')
si.SetProperty3('light2', 'translate', 10, 10, -10)
si.SetProperty3('light2', 'scale', .5, .5, .5)
si.SetProperty1('light2', 'intensity', 1)
si.SetProperty1('light1', 'sample_count', 16)
#Texture
si.NewTexture('tex1', '../mip/pisa.mip')
#Shader
si.NewShader('dome_shader', 'ConstantShader')
si.AssignTexture('dome_shader', 'texture', 'tex1')
si.NewShader('floor_shader', 'PlasticShader')
si.SetProperty3('floor_shader', 'diffuse', .2, .25, .3)
intensity = 0
si.NewShader('sphere_shader', 'PlasticShader')
si.SetProperty3('sphere_shader', 'diffuse', intensity, intensity, intensity)
si.SetProperty1('sphere_shader', 'ior', 40)
#Mesh
si.NewMesh('dome_mesh', '../mesh/dome.mesh')
si.NewMesh('floor_mesh', '../mesh/floor.mesh')
si.NewMesh('sphere_mesh', '../mesh/sphere.mesh')
#ObjectInstance
si.NewObjectInstance('dome1', 'dome_mesh')
si.SetProperty3('dome1', 'rotate', 0, rot, 0)
si.SetProperty3('dome1', 'scale', -3, 3, 3)
si.AssignShader('dome1', 'dome_shader')
rot=rot+10
si.NewObjectInstance('floor1', 'floor_mesh')
si.SetProperty3('floor1', 'scale', 50, 50, 50)
si.AssignShader('floor1', 'floor_shader')
si.NewObjectInstance('sphere1', 'sphere_mesh')
si.AssignShader('sphere1', 'sphere_shader')
si.SetProperty3('sphere1', 'translate', 0, 1, 0)
#si.SetProperty3('sphere1', 'scale', .5, .5, .5)
#ObjectGroup
si.NewObjectGroup('group1')
si.AddObjectToGroup('group1', 'sphere1')
si.AssignObjectGroup('sphere1', 'shadow_target', 'group1')
si.AssignObjectGroup('floor1', 'shadow_target', 'group1')
#FrameBuffer
si.NewFrameBuffer('fb1', 'rgba')
#Renderer
si.NewRenderer('ren1')
si.AssignCamera('ren1', 'cam1')
si.AssignFrameBuffer('ren1', 'fb1')
#si.SetProperty2('ren1', 'resolution', 640, 480)
si.SetProperty2('ren1', 'resolution', 320, 240)
si.SetProperty2('ren1', 'pixelsamples', 12, 12)
#Rendering
si.RenderScene('ren1')
#Output
si.SaveFrameBuffer('fb1', ("../sphere%03d" % f) + ".fb")
#Run commands
si.Run()
#si.Print()
ムービーはこちら
http://rman.sakura.ne.jp/pict/out_1.mp4
出力したイメージを変換して動画にするpythonスクリプト例
#fbpict.py
from subprocess import check_call
for i in range(1,37):
name="sphere"
check_call(["fb2exr", (name+"%03d" % i)+".fb", (name+"%03d" % i)+".exr"])
check_call(["exrtopng", (name+"%03d" % i)+".exr", (name+"%03d" % i)+".png"])
check_call(["ffmpeg", "-i", name+"%03d.png","-s", "320x240" ,"-vcodec", "mjpeg", "-sameq", "out.avi"])
ターミナルを複数起動してフレームごとにレンダリングさせるための
pythonスクリプト例。9スレッドで36フレームをそれぞれ4フレームずつレンダリングした。合計約28分。複数のFujiyama Rendererを立ち上げて実行できます。
あらかじめ、.bashrcにPYTHONPATHを記入しておいた方が良いです。
export PYTHONPATH=$PYTHONPATH:/usr/lib/python2.6/site-packages/:$HOME/Fujiyama-Renderer/bin/
>
#start.py
from subprocess import check_call
check_call(["gnome-terminal", "-e", "python povsphere02a.py"])
check_call(["gnome-terminal", "-e", "python povsphere02b.py"])
check_call(["gnome-terminal", "-e", "python povsphere02c.py"])
check_call(["gnome-terminal", "-e", "python povsphere02d.py"])
check_call(["gnome-terminal", "-e", "python povsphere02e.py"])
check_call(["gnome-terminal", "-e", "python povsphere02f.py"])
check_call(["gnome-terminal", "-e", "python povsphere02g.py"])
check_call(["gnome-terminal", "-e", "python povsphere02h.py"])
check_call(["gnome-terminal", "-e", "python povsphere02i.py"])
ありがとうございます。Pythonの勉強になりますね。
はじめてのFujiyama Renderer その8 視野変換
とりあえず、カメラ位置と注視点位置から視野変換するPythonスクリプトをRenderMan資料placecam.cを参考に求めてみました。ありがとうございます。
まちがっているところもあるかもしれませんが、それっぽくカメラ位置が求められています。
Fujiyama Rendere0.1.1では、変換の順序は、プロパティを設定する順序に
影響しません。記述の順番は関係しません。
順序を設定するためのプロパティは'transform_order'、
'rotate_order'になり、デフォルトではそれぞれ、ORDER_SRTと
ORDER_XYZになっています。
変換はスケール、回転、移動の順に行われています。
回転はx,y,z軸回転の順に行われています。
以下のfujicam.py、Pythonスクリプトを実行し、出力された変換をコピペでシーンに張り付けてください。なおroll、coneangleは未対応です。
#!/usr/bin/env python
#fujicam.py
import sys
import os
from math import *
PI=3.1415926535897932
def usage():
print "usage: placecam pos_x pos_y pos_z aim_x aim_y aim_z"
print " [coneangle] [roll_angle]"
print " Calculate Fujiyama Renderer transforms needed for camera transform"
print " from light position to aim point with the given roll angle."
sys.exit(1)
def RiRotate(xangle, yangle, zangle):
if (fabs(xangle) > 0.001 or fabs(yangle) > 0.001 or fabs(zangle) > 0.001):
print "rotate %0.2f %0.2f %0.2f" % (xangle, yangle, zangle)
def RiTranslate(dx, dy, dz):
print "translate %0.2f %0.2f %0.2f" % (dx, dy, dz)
"""
/*
* AimZ(): rotate the world so the direction vector points in
* positive z by rotating about the y axis, then x. The cosine
* of each rotation is given by components of the normalized
* direction vector. Before the y rotation the direction vector
* might be in negative z, but not afterward.
*/
"""
def AimZ(direction):
global PI
if (direction[0]==0 and direction[1]==0 and direction[2]==0):
return
#/*
# * The initial rotation about the y axis is given by the projection of
# * the direction vector onto the x,z plane: the x and z components
# * of the direction.
# */
xzlen = sqrt(direction[0]*direction[0]+direction[2]*direction[2])
if (xzlen == 0):
if (direction[1] < 0):
yrot = 180
else:
yrot=0
else:
yrot = 180-180*acos(direction[2]/xzlen)/PI
# /*
# * The second rotation, about the x axis, is given by the projection on
# * the y,z plane of the y-rotated direction vector: the original y
# * component, and the rotated x,z vector from above.
# */
yzlen = sqrt(direction[1]*direction[1]+xzlen*xzlen)
xrot = 180*acos(xzlen/yzlen)/PI; #/* yzlen should never be 0 */
if (direction[1] > 0):
RiRotate(xrot, 0.0, 0.0)
else:
RiRotate(-xrot, 0.0, 0.0)
# /* The last rotation declared gets performed first */
if (direction[0] > 0):
RiRotate( 0.0, -yrot, 0.0)
else:
RiRotate( 0.0, yrot, 0.0)
def PlaceCamera(position, direction, roll):
RiRotate(0.0, 0.0, -roll)
AimZ(direction)
RiTranslate(position[0], position[1], position[2])
def main():
global PI
if len(sys.argv) < 7:
usage()
pos=[0,0,0]
aim=[0,0,0]
dir=[0,0,0]
pos[0] = float(sys.argv[1])
pos[1] = float(sys.argv[2])
pos[2] = float(sys.argv[3])
aim[0] = float(sys.argv[4])
aim[1] = float(sys.argv[5])
aim[2] = float(sys.argv[6])
if len(sys.argv) > 7:
coneangle = float(sys.argv[7])
else:
coneangle = 0.0
if len(sys.argv) > 8:
roll = float(sys.argv[8])
else:
roll = 0.0
print "position: %0.2f, %0.2f, %0.2f"% (pos[0], pos[1], pos[2])
print "aim: %0.2f, %0.2f, %0.2f"% (aim[0], aim[1], aim[2])
print "coneangle: %0.4f"% coneangle
print "roll: %0.2f\n" % roll
if (coneangle != 0.0):
fov = coneangle * 360.0 / PI
print "Projection \"perspective\" \"fov\" [%0.2f]" % fov
dir[0] = aim[0] - pos[0]
dir[1] = aim[1] - pos[1]
dir[2] = aim[2] - pos[2]
PlaceCamera(pos, dir, roll)
if __name__ == "__main__":
main()
いろいろと試したくなります。ありがとうございます。
2013/8/24 : coneangle箇所修正
はじめてのFujiyama Renderer その9
外部モジュールを作成した。
以下、colorinc.py 未完成ですが、いろいろやってみる。
#! /usr/bin/env python
#colorinc.py
import fujiyama
si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
si.OpenPlugin('GlassShader')
si.NewShader('Blue_shader', 'PlasticShader')
si.SetProperty3('Blue_shader', 'diffuse', 0, 0.6, 1)
si.NewShader('Red_shader', 'PlasticShader')
si.SetProperty3('Red_shader', 'diffuse', 1, 0, 0)
si.NewShader('Green_shader', 'PlasticShader')
si.SetProperty3('Green_shader', 'diffuse', 0, 1, 0.5)
si.NewShader('Violet_shader', 'PlasticShader')
si.SetProperty3('Violet_shader', 'diffuse', 1, 0.2, 1)
si.NewShader('Orange_shader', 'PlasticShader')
si.SetProperty3('Orange_shader', 'diffuse', 1, 0.6, 0.2)
si.NewShader('Yellow_shader', 'PlasticShader')
si.SetProperty3('Yellow_shader', 'diffuse', 1,1,0)
si.NewShader('Cyan_shader', 'PlasticShader')
si.SetProperty3('Cyan_shader', 'diffuse', 0, 1, 1)
si.NewShader('Magenta_shader', 'PlasticShader')
si.SetProperty3('Magenta_shader', 'diffuse', 1, 0, 1)
si.NewShader('White_shader', 'PlasticShader')
si.SetProperty3('White_shader', 'diffuse', 1, 1, 1)
si.NewShader('Black_shader', 'PlasticShader')
si.SetProperty3('Black_shader', 'diffuse', 0, 0, 0)
si.NewShader('DimGray_shader', 'PlasticShader')
si.SetProperty3('DimGray_shader', 'diffuse',0.329412,0.329412,0.329412)
si.NewShader('Gray_shader', 'PlasticShader')
si.SetProperty3('Gray_shader', 'diffuse',0.752941,0.752941,0.752941)
si.NewShader('LightGray_shader', 'PlasticShader')
si.SetProperty3('LightGray_shader', 'diffuse',0.658824,0.658824,0.658824)
si.NewShader('VLightGray_shader', 'PlasticShader')
si.SetProperty3('VLightGray_shader', 'diffuse',0.80,0.80,0.80)
si.NewShader('Aquamarine_shader', 'PlasticShader')
si.SetProperty3('Aquamarine_shader', 'diffuse',0.439216,0.858824,0.576471)
si.NewShader('BlueViolet_shader', 'PlasticShader')
si.SetProperty3('BlueViolet_shader', 'diffuse',0.62352,0.372549,0.623529)
si.NewShader('Brown_shader', 'PlasticShader')
si.SetProperty3('Brown_shader', 'diffuse',0.647059,0.164706,0.164706)
si.NewShader('CadetBlue_shader', 'PlasticShader')
si.SetProperty3('CadetBlue_shader', 'diffuse',0.372549,0.623529,0.623529)
si.NewShader('Coral_shader', 'PlasticShader')
si.SetProperty3('Coral_shader', 'diffuse',1.0,0.498039,0.0)
si.NewShader('CornflowerBlue_shader', 'PlasticShader')
si.SetProperty3('CornflowerBlue_shader', 'diffuse',0.258824,0.258824,0.435294)
si.NewShader('DarkGreen_shader', 'PlasticShader')
si.SetProperty3('DarkGreen_shader', 'diffuse',0.184314,0.309804,0.184314)
si.NewShader('DarkOliveGreen_shader', 'PlasticShader')
si.SetProperty3('DarkOliveGreen_shader', 'diffuse',0.309804,0.309804,0.184314)
si.NewShader('DarkOrchid_shader', 'PlasticShader')
si.SetProperty3('DarkOrchid_shader', 'diffuse',0.6,0.196078,0.8)
si.NewShader('DarkSlateBlue_shader', 'PlasticShader')
si.SetProperty3('DarkSlateBlue_shader', 'diffuse',0.119608,0.137255,0.556863)
si.NewShader('DarkSlateGray_shader', 'PlasticShader')
si.SetProperty3('DarkSlateGray_shader', 'diffuse',0.184314,0.309804,0.309804)
si.NewShader('DarkTurquoise_shader', 'PlasticShader')
si.SetProperty3('DarkTurquoise_shader', 'diffuse',0.439216,0.576471,0.858824)
si.NewShader('Firebrick_shader', 'PlasticShader')
si.SetProperty3('Firebrick_shader', 'diffuse',0.556863,0.137255,0.137255)
si.NewShader('ForestGreen_shader', 'PlasticShader')
si.SetProperty3('ForestGreen_shader', 'diffuse',0.137255,0.556863,0.137255)
si.NewShader('Gold_shader', 'PlasticShader')
si.SetProperty3('Gold_shader', 'diffuse',0.8,0.498039,0.196078)
si.NewShader('Goldenrod_shader', 'PlasticShader')
si.SetProperty3('Goldenrod_shader', 'diffuse',0.858824,0.858824,0.439216)
si.NewShader('GreenYellow_shader', 'PlasticShader')
si.SetProperty3('GreenYellow_shader', 'diffuse',0.576471,0.858824,0.439216)
si.NewShader('IndianRed_shader', 'PlasticShader')
si.SetProperty3('IndianRed_shader', 'diffuse',0.309804,0.184314,0.184314)
si.NewShader('Khaki_shader', 'PlasticShader')
si.SetProperty3('Khaki_shader', 'diffuse',0.623529,0.623529,0.372549)
"""
LightBlue=vector3(0.74902,0.847059,0.847059)
LightSteelBlue=vector3(0.560784,0.560784,0.737255)
LimeGreen=vector3(0.196078,0.8,0.196078)
Maroon=vector3(0.556863,0.137255,0.419608)
MediumAquamarine=vector3(0.196078,0.8,0.6)
MediumBlue=vector3(0.196078,0.196078,0.8)
MediumForestGreen=vector3(0.419608,0.556863,0.137255)
MediumGoldenrod=vector3(0.917647,0.917647,0.678431)
MediumOrchid=vector3(0.576471,0.439216,0.858824)
MediumSeaGreen=vector3(0.258824,0.435294,0.258824)
MediumTurquoise=vector3(0.439216,0.858824,0.858824)
MediumVioletRed=vector3(0.858824,0.439216,0.576471)
MidnightBlue=vector3(0.184314,0.184314,0.309804)
Navy=vector3(0.137255,0.137255,0.556863)
NavyBlue=vector3(0.137255,0.137255,0.556863)
Orange=vector3(1,0.5,0.0)
Orchid=vector3(0.858824,0.439216,0.858824)
PaleGreen=vector3(0.560784,0.737255,0.560784)
Pink=vector3(0.737255,0.560784,0.560784)
Plum=vector3(0.917647,0.678431,0.917647)
Salmon=vector3(0.435294,0.258824,0.258824)
SeaGreen=vector3(0.137255,0.556863,0.419608)
Sienna=vector3(0.556863,0.419608,0.137255)
SkyBlue=vector3(0.196078,0.6,0.8)
SteelBlue=vector3(0.137255,0.419608,0.556863)
Tan=vector3(0.858824,0.576471,0.439216)
Thistle=vector3(0.847059,0.74902,0.847059)
Turquoise=vector3(0.678431,0.917647,0.917647)
Violet=vector3(0.309804,0.184314,0.309804)
VioletRed=vector3(0.8,0.196078,0.6)
Wheat=vector3(0.847059,0.847059,0.74902)
YellowGreen=vector3(0.6,0.8,0.196078)
SummerSky=vector3(0.22,0.69,0.87)
RichBlue=vector3(0.35,0.35,0.67)
Brass=vector3( 0.71,0.65,0.26)
Copper=vector3(0.72,0.45,0.20)
Bronze=vector3(0.55,0.47,0.14)
Bronze2=vector3(0.65,0.49,0.24)
Silver=vector3(0.90,0.91,0.98)
BrightGold=vector3(0.85,0.85,0.10)
OldGold=vector3( 0.81,0.71,0.23)
Feldspar=vector3(0.82,0.57,0.46)
Quartz=vector3(0.85,0.85,0.95)
NeonPink=vector3(1.00,0.43,0.78)
DarkPurple=vector3(0.53,0.12,0.47)
NeonBlue=vector3(0.30,0.30,1.00)
CoolCopper=vector3(0.85,0.53,0.10)
MandarinOrange=vector3(0.89,0.47,0.20)
LightWood=vector3(0.91,0.76,0.65)
MediumWood=vector3(0.65,0.50,0.39)
DarkWood=vector3(0.52,0.37,0.26)
SpicyPink=vector3(1.00,0.11,0.68)
SemiSweetChoc=vector3(0.42,0.26,0.15)
BakersChoc=vector3(0.36,0.20,0.09)
Flesh=vector3(0.96,0.80,0.69)
NewTan=vector3(0.92,0.78,0.62)
NewMidnightBlue=vector3(0.00,0.00,0.61)
VeryDarkBrown=vector3(0.35,0.16,0.14)
DarkBrown=vector3(0.36,0.25,0.20)
DarkTan=vector3(0.59,0.41,0.31)
GreenCopper=vector3(0.32,0.49,0.46)
DkGreenCopper=vector3(0.29,0.46,0.43)
DustyRose=vector3(0.52,0.39,0.39)
HuntersGreen=vector3(0.13,0.37,0.31)
Scarlet=vector3(0.55,0.09,0.09)
Med_Purple=vector3( 0.73,0.16,0.96)
Light_Purple=vector3(0.87,0.58,0.98)
Very_Light_Purple=vector3(0.94,0.81,0.99)
"""
さらにcolorinc.pyをインポートするシーン例
#!/usr/bin/env python
# -*- coding: utf-8 -*-
#export PYTHONPATH=$PYTHONPATH:/usr/lib/python2.6/site-packages/:$HOME/Fujiyama-Renderer/bin/
from colorinc import si
import fujiyama
#si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
si.OpenPlugin('GlassShader')
#Camera
si.NewCamera('cam1', 'PerspectiveCamera')
si.SetProperty3('cam1', 'rotate', -26.57, 0.00, 0.00)
#si.SetProperty3('cam1', 'rotate', 0.00, 180.00, 0.00)
si.SetProperty3('cam1', 'translate', 0.00, 5.00, 10.00)
#Light
"""
si.NewLight('lighta', 'PointLight')
si.SetProperty3('lighta', 'translate', -5, 4, 5)
si.SetProperty1('lighta', 'intensity', 0.03)
si.NewLight('lightb', 'SphereLight')
si.SetProperty3('lightb', 'translate', 5, 4, 5)
si.SetProperty3('lightb', 'scale', .5, .5, .5)
si.SetProperty1('lightb', 'intensity', 0.03)
"""
#Light
si.NewLight( 'light0', 'PointLight')
si.SetProperty3( 'light0', 'translate', 0.900771, 12, 4.09137)
si.SetProperty1( 'light0', 'intensity', 0.03125)
si.NewLight( 'light1', 'PointLight')
si.SetProperty3( 'light1', 'translate', 2.02315, 12, 5.28021)
si.SetProperty1( 'light1' ,'intensity', 0.03125)
si.NewLight( 'light2', 'PointLight')
si.SetProperty3( 'light2', 'translate', 10.69, 12, 13.918)
si.SetProperty1( 'light2', 'intensity', 0.03125)
si.NewLight( 'light3', 'PointLight')
si.SetProperty3( 'light3', 'translate', 4.28027, 12, 7.58462)
si.SetProperty1( 'light3', 'intensity', 0.03125)
si.NewLight( 'light4', 'PointLight')
si.SetProperty3( 'light4', 'translate', 12.9548, 12, 1.19914)
si.SetProperty1( 'light4', 'intensity', 0.03125)
si.NewLight( 'light5', 'PointLight')
si.SetProperty3( 'light5', 'translate', 6.55808, 12, 2.31772)
si.SetProperty1( 'light5', 'intensity', 0.03125)
si.NewLight( 'light6', 'PointLight')
si.SetProperty3( 'light6', 'translate', 0.169064, 12, 10.9623)
si.SetProperty1( 'light6', 'intensity', 0.03125)
si.NewLight( 'light7', 'PointLight')
si.SetProperty3( 'light7', 'translate', 1.25002, 12, 4.51314)
si.SetProperty1( 'light7', 'intensity', 0.03125)
si.NewLight( 'light8', 'PointLight')
si.SetProperty3( 'light8', 'translate', 2.46758, 12, 5.73382)
si.SetProperty1( 'light8', 'intensity', 0.03125)
si.NewLight( 'light9', 'PointLight')
si.SetProperty3( 'light9', 'translate', 3.55644, 12, 6.84334)
si.SetProperty1( 'light9','intensity', 0.03125)
si.NewLight( 'light10', 'PointLight')
si.SetProperty3( 'light10', 'translate', 4.76112, 12, 8.00264)
si.SetProperty1( 'light10', 'intensity', 0.03125)
si.NewLight( 'light11', 'PointLight')
si.SetProperty3( 'light11', 'translate', 13.3267,12, 9.10333)
si.SetProperty1( 'light11', 'intensity', 0.03125)
si.NewLight( 'light12', 'PointLight')
si.SetProperty3( 'light12', 'translate', 14.4155, 12, 2.68084)
si.SetProperty1( 'light12', 'intensity', 0.03125)
si.NewLight( 'light13', 'PointLight')
si.SetProperty3( 'light13', 'translate', 8.10755, 12, 3.79629)
si.SetProperty1( 'light13', 'intensity', 0.03125)
si.NewLight( 'light14', 'PointLight')
si.SetProperty3( 'light14', 'translate', 9.21103, 12, 4.9484)
si.SetProperty1( 'light14', 'intensity', 0.03125)
si.NewLight( 'light15', 'PointLight')
si.SetProperty3( 'light15', 'translate', 2.83469, 12, 6.09221)
si.SetProperty1( 'light15', 'intensity', 0.03125)
si.NewLight( 'light16', 'PointLight')
si.SetProperty3( 'light16', 'translate', 4.00945, 12, 7.18302)
si.SetProperty1( 'light16', 'intensity', 0.03125)
si.NewLight( 'light17', 'PointLight')
si.SetProperty3( 'light17', 'translate', 12.6072, 12, 0.832089)
si.SetProperty1( 'light17', 'intensity', 0.03125)
si.NewLight( 'light18', 'PointLight')
si.SetProperty3( 'light18', 'translate', 6.21169, 12, 1.98055)
si.SetProperty1( 'light18', 'intensity', 0.03125)
si.NewLight( 'light19', 'PointLight')
si.SetProperty3( 'light19', 'translate', 7.39599, 12, 10.5563)
si.SetProperty1( 'light19', 'intensity', 0.03125)
si.NewLight( 'light20', 'PointLight')
si.SetProperty3( 'light20', 'translate', 8.52421, 12, 4.15086)
si.SetProperty1( 'light20', 'intensity', 0.03125)
si.NewLight( 'light21', 'PointLight')
si.SetProperty3( 'light21', 'translate', 9.5891, 12, 5.39715)
si.SetProperty1( 'light21', 'intensity', 0.03125)
si.NewLight( 'light22', 'PointLight')
si.SetProperty3( 'light22', 'translate', 3.18967, 12, 13.9542)
si.SetProperty1( 'light22', 'intensity', 0.03125)
si.NewLight( 'light23','PointLight')
si.SetProperty3( 'light23', 'translate', 4.41432, 12, 0.082813)
si.SetProperty1( 'light23', 'intensity', 0.03125)
si.NewLight( 'light24', 'PointLight')
si.SetProperty3( 'light24', 'translate', 5.48803, 12, 1.21856)
si.SetProperty1( 'light24', 'intensity', 0.03125)
si.NewLight( 'light25', 'PointLight')
si.SetProperty3( 'light25', 'translate', 6.57647, 12, 2.31432)
si.SetProperty1( 'light25', 'intensity', 0.03125)
si.NewLight( 'light26', 'PointLight')
si.SetProperty3( 'light26', 'translate', 0.265098, 12, 10.9453)
si.SetProperty1( 'light26', 'intensity', 0.03125)
si.NewLight( 'light27', 'PointLight')
si.SetProperty3( 'light27', 'translate', 8.84422, 12, 12.1117)
si.SetProperty1( 'light27', 'intensity', 0.03125)
si.NewLight( 'light28', 'PointLight')
si.SetProperty3( 'light28', 'translate', 10.0154, 12, 5.67625)
si.SetProperty1( 'light28', 'intensity', 0.03125)
si.NewLight( 'light29', 'PointLight')
si.SetProperty3( 'light29', 'translate', 11.0907, 12, 14.4043)
si.SetProperty1( 'light29', 'intensity', 0.03125)
si.NewLight( 'light30', 'PointLight')
si.SetProperty3( 'light30', 'translate', 4.71726, 12, 7.98851)
si.SetProperty1( 'light30', 'intensity', 0.03125)
si.NewLight( 'light31', 'PointLight')
si.SetProperty3( 'light31', 'translate', 13.3907, 12, 9.08986)
si.SetProperty1( 'light31', 'intensity', 0.03125)
#Shader
si.NewShader('floor_shader', 'PlasticShader')
si.SetProperty3('floor_shader', 'diffuse', .2, .25, .3)
si.SetProperty1('floor_shader', 'ior', 2)
si.NewShader('dome_shader', 'ConstantShader')
si.SetProperty3('dome_shader', 'diffuse', .8, .8, .8)
#Mesh
si.NewMesh('dome_mesh', '../mesh/dome.mesh')
si.NewMesh('floor_mesh', '../mesh/floor.mesh')
si.NewMesh('cube_mesh', '../mesh/cube.mesh')
#ObjectInstance
si.NewObjectInstance('cube1', 'cube_mesh')
si.AssignShader('cube1', 'DarkTurquoise_shader')
si.SetProperty3('cube1', 'translate', 0,0, 0)
si.NewObjectInstance('cube2', 'cube_mesh')
si.AssignShader('cube2', 'Firebrick_shader')
si.SetProperty3('cube2', 'translate', -2,0, 0)
si.NewObjectInstance('cube3', 'cube_mesh')
si.AssignShader('cube3', 'ForestGreen_shader')
si.SetProperty3('cube3', 'translate', 2,0, 0)
si.SetProperty3('cube3', 'rotate', 0,45, 0)
si.NewObjectInstance('cube4', 'cube_mesh')
si.AssignShader('cube4', 'Gold_shader')
si.SetProperty3('cube4', 'translate', -2,0, -2)
si.NewObjectInstance('cube5', 'cube_mesh')
si.AssignShader('cube5', 'Goldenrod_shader')
si.SetProperty3('cube5', 'translate', 0,0, -2)
si.NewObjectInstance('cube6', 'cube_mesh')
si.AssignShader('cube6', 'GreenYellow_shader')
si.SetProperty3('cube6', 'translate', 2,0, -2)
si.NewObjectInstance('floor1', 'floor_mesh')
si.AssignShader('floor1', 'Blue_shader')
si.SetProperty3('Blue_shader', 'reflect', 0.5, 0.5, 0.5)
si.SetProperty3('floor1', 'translate', 0,-0.5, 0)
si.SetProperty3('floor1', 'scale', 10, 10, 10)
si.NewObjectInstance('dome1', 'dome_mesh')
si.AssignShader('dome1', 'dome_shader')
#FrameBuffer
si.NewFrameBuffer('fb1', 'rgba')
#Properties
si.ShowPropertyList('Renderer')
#Renderer
si.NewRenderer('ren1')
si.AssignCamera('ren1', 'cam1')
si.AssignFrameBuffer('ren1', 'fb1')
si.SetProperty2('ren1', 'resolution', 512, 300)
#si.SetProperty2('ren1', 'resolution', 160, 120)
si.SetProperty1('ren1', 'raymarch_step', .01)
si.SetProperty1('ren1', 'raymarch_shadow_step', .02)
si.SetProperty1('ren1', 'raymarch_reflect_step', .02)
#Rendering
si.RenderScene('ren1')
#Output
si.SaveFrameBuffer('fb1', '../boxcolor01.fb')
#Run commands
si.Run()
#si.Print()
カメラの画角調整 20度
si.SetProperty1('cam1', 'fov', 20)
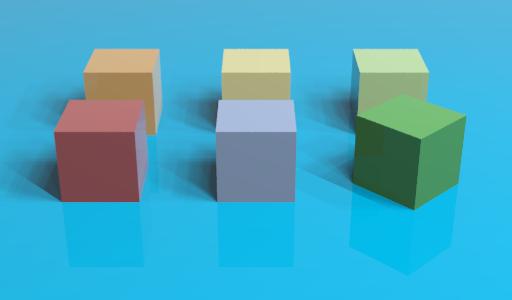
ありがとうございます。
はじめてのFujiyama Renderer その10
Thank you for your support.
#!/usr/bin/env python
# 1 happy with 1 dome light with an HDRI
# Copyright (c) 2011-2013 Hiroshi Tsubokawa
from colorinc import si
import fujiyama
#si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
#Camera
si.NewCamera('cam1', 'PerspectiveCamera')
si.SetSampleProperty3('cam1', 'translate', 0, 1, 8.5, 0)
rot = 120
#Light
si.NewLight('light1', 'DomeLight')
si.SetProperty3('light1', 'rotate', 0, rot, 0)
si.SetProperty1('light1', 'sample_count', 256)
#Texture
si.NewTexture('tex1', '../mip/pisa.mip')
si.AssignTexture('light1', 'environment_map', 'tex1');
#Shader
si.NewShader('happy_shader', 'PlasticShader')
si.SetProperty3('happy_shader', 'diffuse', .8, .8, .8)
si.NewShader('dome_shader', 'ConstantShader')
si.AssignTexture('dome_shader', 'texture', 'tex1')
si.NewShader('sphere_shader1', 'PlasticShader')
si.SetProperty3('sphere_shader1', 'diffuse', 0, 0, 0)
si.SetProperty1('sphere_shader1', 'ior', 40)
si.NewShader('sphere_shader2', 'PlasticShader')
si.SetProperty3('sphere_shader2', 'diffuse', 1, 1, 1)
si.SetProperty3('sphere_shader2', 'reflect', 0, 0, 0)
si.NewShader('floor_shader', 'PlasticShader')
#si.SetProperty3('floor_shader', 'diffuse', .8, .85, .8)
#si.SetProperty3('floor_shader', 'reflect', 0, 0, 0)
#Mesh
#si.NewMesh('happy_mesh', '../../mesh/happy.mesh')
si.NewMesh('dome_mesh', '../mesh/dome.mesh')
si.NewMesh('sphere_mesh', '../mesh/sphere.mesh')
si.NewMesh('floor_mesh', '../mesh/floor.mesh')
#ObjectInstance
#si.NewObjectInstance('happy1', 'happy_mesh')
#si.AssignShader('happy1', 'happy_shader')
si.NewObjectInstance('floor1', 'floor_mesh')
si.AssignShader('floor1', 'floor_shader')
si.SetProperty3('floor_shader', 'reflect', 0, 0, 0)
si.NewObjectInstance('dome1', 'dome_mesh')
si.SetProperty3('dome1', 'rotate', 0, rot, 0)
si.SetProperty3('dome1', 'scale', -.5, .5, .5)
si.AssignShader('dome1', 'dome_shader')
si.NewObjectInstance('sphere1', 'sphere_mesh')
si.AssignShader('sphere1', 'sphere_shader1')
si.SetProperty3('sphere1', 'translate', -1.5, .52, 0)
si.SetProperty3('sphere1', 'scale', .5, .53, .5)
si.NewObjectInstance('sphere2', 'sphere_mesh')
si.AssignShader('sphere2', 'Blue_shader')
si.SetProperty3('sphere2', 'translate', 1.5, .52, 0)
si.SetProperty3('sphere2', 'scale', .5, .53, .5)
#ObjectGroup
#si.NewObjectGroup('group1')
#si.AddObjectToGroup('group1', 'happy1')
#si.AssignObjectGroup('happy1', 'shadow_target', 'group1')
si.NewObjectGroup('group2')
#si.AddObjectToGroup('group2', 'floor1')
si.AddObjectToGroup('group2', 'sphere1')
si.AddObjectToGroup('group2', 'sphere2')
si.AssignObjectGroup('sphere1', 'shadow_target', 'group2')
si.AssignObjectGroup('sphere2', 'shadow_target', 'group2')
si.AssignObjectGroup('floor1', 'shadow_target', 'group2')
si.NewObjectGroup('group3')
si.AddObjectToGroup('group3', 'dome1')
si.AssignObjectGroup('sphere1', 'reflect_target', 'group3')
si.NewObjectGroup('group4')
si.AddObjectToGroup('group4', 'dome1')
#si.AddObjectToGroup('group4', 'happy1')
#si.AssignObjectGroup('happy1', 'reflect_target', 'group4')
#FrameBuffer
si.NewFrameBuffer('fb1', 'rgba')
#Renderer
si.NewRenderer('ren1')
si.AssignCamera('ren1', 'cam1')
si.AssignFrameBuffer('ren1', 'fb1')
si.SetProperty2('ren1', 'resolution', 640, 480)
#si.SetProperty2('ren1', 'resolution', 160, 120)
#si.SetProperty2('ren1', 'pixelsamples', 9, 9)
#Rendering
si.RenderScene('ren1')
#Output
si.SaveFrameBuffer('fb1', '../dome_floor1.fb')
#Run commands
si.Run()
#si.Print()
はじめてのFujiyama Renderer その11
python cgkitを使ってFujiyama Rendererのサンプル内の32個のPointLightの位置を確認してみました。
cgkitのviewer.pyで確認したところ、四角形の対角になるような斜め配置になっていました。
cgkitのrender.pyを使ってレンダリング確認、画像は3Delightで生成しました。
# -*- coding: shift-jis -*-
# 日本語のコメント挿入
import camctrl
Globals( rib='Imager "background" "color background" [0.2 0.4 0.6]',
up = (0,1,0),
handedness = 'l'
)
"""
TargetCamera(
pos = (0,0,-10),
target = (0,0,0)
)
"""
TargetCamera(
name = 'TargetCamera',
pos = (14.5232, 22.6823, -19.6213),
target = (5.26602, 4.49559, 6.54843),
fov = 45.000000,
roll = 0.000000,
focallength = 0.000000,
fstop = 0.000000,
auto_nearfar = True,
nearplane = 0.100000,
farplane = 1000.000000,
)
SpotLight3DS( name = "SpotLight3DS",
enabled = True,
intensity = 1.4,
shadowed = True,
shadow_size = 1024,
shadow_filter = 8.0,
shadow_bias = 0.001,
hotspot = 80,
falloff = 90,
pos=(-10, 10, -20),
target = (0,0,0) )
"""
GLPointLight(
pos = (-10,3, -20),
diffuse = (1, 1, 1)
)
"""
# ライト位置にBox
Box(
lx = 0.1,
ly = 0.1,
lz = 0.1,
pos = (-10,9, -20),
material = GLMaterial(diffuse=(1, 0.7, 0.2))
)
#PointLight Position
listlight=[
( 0.900771, 12, 4.09137),
( 2.02315, 12, 5.28021),
( 10.69, 12, 13.918),
( 4.28027, 12, 7.58462),
( 12.9548, 12, 1.19914),
( 6.55808, 12, 2.31772),
( 0.169064, 12, 10.9623),
( 1.25002, 12, 4.51314),
( 2.46758, 12, 5.73382),
( 3.55644, 12, 6.84334),
( 4.76112, 12, 8.00264),
( 13.3267,12, 9.10333),
( 14.4155, 12, 2.68084),
( 8.10755, 12, 3.79629),
( 9.21103, 12, 4.9484),
( 2.83469, 12, 6.09221),
( 4.00945, 12, 7.18302),
(12.6072, 12, 0.832089),
( 6.21169, 12, 1.98055),
( 7.39599, 12, 10.5563),
( 8.52421, 12, 4.15086),
( 9.5891, 12, 5.39715),
( 3.18967, 12, 13.9542),
( 4.41432, 12, 0.082813),
( 5.48803, 12, 1.21856),
( 6.57647, 12, 2.31432),
( 0.265098, 12, 10.9453),
( 8.84422, 12, 12.1117),
( 10.0154, 12, 5.67625),
(11.0907, 12, 14.4043),
( 4.71726, 12, 7.98851),
( 13.3907, 12, 9.08986),
]
for i in range(32):
Sphere(
radius = 0.2,
pos = listlight[i],
material = GLMaterial(diffuse = (0, 0.6, 1)))
# Boxの表示
Box(
lx = 4,
ly = 4,
lz = 4,
pos = (0,0,1),
rot = mat3().fromEulerYXZ(radians(-30), radians(20), 0),
material = GLMaterial(diffuse=(1,0,0))
)
Plane(
name = "Plane",
lx = 10,
ly = 10,
pos = (0,-3,0),
rot = mat3().fromEulerYXZ(radians(-90),0, 0),
scale = vec3(10,10,10),
material =GLMaterial(diffuse=(1,1,1))
)
POV-Rayのdefault cameraのangleを求める
以下、Pov-rayでは未検証ですが、計算してみました。
パースペクティブ・カメラの主なディフォルト設定(省略するとこの設定値が使用されている。)
camera {
perspective
location <0,0,0>
direction <0,0,1>
right <1.33,0,0>
sky <0,1,0>
up <0,1,0>
look_at <0,0,1>
}
画角(angle)
angle、right、directionの関係は次式で表される(right_lengthとdirection_lengthはそれぞれrightとdirectionベクトルの長さ)。
direction_length = 0.5 * right_length / tan(angle / 2)
デフォルトカメラのangleは、
1=0.5*1.33/ tan(angle / 2)
tan(angle / 2)=0.5*1.33
angle / 2=atan(0.5*1.33)
angle=2*atan(0.5*1.33)
で求められるので、以下python cgkitで求めてみました。
>python
Python 2.6.5 (r265:79096, Mar 19 2010, 18:02:59) [MSC v.1500 64 bit (AMD64)
win32 Type "help", "copyright", "credits" or "license" for more information.
>>> from cgkit.all import *
>>> right=vec3(1.33,0,0)
>>> right.length()
1.3300000000000001
>>> direction=vec3(0,0,1)
>>> direction.length()
1.0
>>> import math
コサイン60度を求めてみる。1/2になるかな。
>>> math.cos(math.radians(60))
0.50000000000000011
アークタンジェント:atan(x) を求める。
>>> 2*math.atan(1.33*0.5)
1.1736957392994543
ラジアンを度に変換する。
>>> math.degrees(2*math.atan(1.33*0.5))
67.247812294345678
>>> angle=math.degrees(2*math.atan(1.33*0.5))
>>> angle
67.247812294345678
ということで67度となりましたが、あっているかどうかは
実際POV-RAYで検証しなくてはなりません。
Fujiyama Renderer dome light with HDRI
HDRIを使うdomelightを試してみた。
120フレームをレンダリングするために12ファイル作り12スレッドで各ファイルは10フレームレンダリングする。レンダリング時間は約40分でした。
以下、
ムービーはこちら
http://rman.sakura.ne.jp/pict/dome_1.mp4
面白いですね。ありがとうございます。
#!/usr/bin/env python
import fujiyama
rot = 0
for f in range(1,11):
si = fujiyama.SceneInterface()
#plugins
si.OpenPlugin('ConstantShader')
si.OpenPlugin('PlasticShader')
#Camera
si.NewCamera('cam1', 'PerspectiveCamera')
si.SetSampleProperty3('cam1', 'translate', 0, 1, 8.5, 0)
si.SetProperty1('cam1', 'fov', 30)
#Light
si.NewLight('light1', 'DomeLight')
si.SetProperty3('light1', 'rotate', 0, rot, 0)
si.SetProperty1('light1', 'sample_count', 256)
rot=rot+3
#Texture
si.NewTexture('tex1', '../mip/pisa.mip')
si.AssignTexture('light1', 'environment_map', 'tex1')
#Shader
si.NewShader('happy_shader', 'PlasticShader')
si.SetProperty3('happy_shader', 'diffuse', .8, .8, .8)
si.NewShader('dome_shader', 'ConstantShader')
si.AssignTexture('dome_shader', 'texture', 'tex1')
si.NewShader('sphere_shader1', 'PlasticShader')
si.SetProperty3('sphere_shader1', 'diffuse', 0, 0, 0)
si.SetProperty1('sphere_shader1', 'ior', 40)
si.NewShader('sphere_shader2', 'PlasticShader')
si.SetProperty3('sphere_shader2', 'diffuse', 0, 0.6, 1)
si.SetProperty3('sphere_shader2', 'reflect', 0, 0, 0)
si.NewShader('floor_shader', 'PlasticShader')
#si.SetProperty3('floor_shader', 'diffuse', .8, .85, .8)
#si.SetProperty3('floor_shader', 'reflect', 0, 0, 0)
#Mesh
#si.NewMesh('happy_mesh', '../../mesh/happy.mesh')
si.NewMesh('dome_mesh', '../mesh/dome.mesh')
si.NewMesh('sphere_mesh', '../mesh/sphere.mesh')
si.NewMesh('floor_mesh', '../mesh/floor.mesh')
#ObjectInstance
#si.NewObjectInstance('happy1', 'happy_mesh')
#si.AssignShader('happy1', 'happy_shader')
si.NewObjectInstance('floor1', 'floor_mesh')
si.AssignShader('floor1', 'floor_shader')
si.SetProperty3('floor_shader', 'reflect', 0, 0, 0)
si.NewObjectInstance('dome1', 'dome_mesh')
si.SetProperty3('dome1', 'rotate', 0, rot, 0)
si.SetProperty3('dome1', 'scale', -.5, .5, .5)
si.AssignShader('dome1', 'dome_shader')
si.NewObjectInstance('sphere1', 'sphere_mesh')
si.AssignShader('sphere1', 'sphere_shader1')
si.SetProperty3('sphere1', 'translate', -1.5, .52, 0)
si.SetProperty3('sphere1', 'scale', .5, .53, .5)
si.NewObjectInstance('sphere2', 'sphere_mesh')
si.AssignShader('sphere2', 'sphere_shader2')
si.SetProperty3('sphere2', 'translate', 1.5, .52, 0)
si.SetProperty3('sphere2', 'scale', .5, .53, .5)
#ObjectGroup
#si.NewObjectGroup('group1')
#si.AddObjectToGroup('group1', 'happy1')
#si.AssignObjectGroup('happy1', 'shadow_target', 'group1')
si.NewObjectGroup('group2')
#si.AddObjectToGroup('group2', 'floor1')
si.AddObjectToGroup('group2', 'sphere1')
si.AddObjectToGroup('group2', 'sphere2')
si.AssignObjectGroup('sphere1', 'shadow_target', 'group2')
si.AssignObjectGroup('sphere2', 'shadow_target', 'group2')
si.AssignObjectGroup('floor1', 'shadow_target', 'group2')
si.NewObjectGroup('group3')
si.AddObjectToGroup('group3', 'dome1')
si.AssignObjectGroup('sphere1', 'reflect_target', 'group3')
si.NewObjectGroup('group4')
si.AddObjectToGroup('group4', 'dome1')
#si.AddObjectToGroup('group4', 'happy1')
#si.AssignObjectGroup('happy1', 'reflect_target', 'group4')
#FrameBuffer
si.NewFrameBuffer('fb1', 'rgba')
#Renderer
si.NewRenderer('ren1')
si.AssignCamera('ren1', 'cam1')
si.AssignFrameBuffer('ren1', 'fb1')
#si.SetProperty2('ren1', 'resolution', 640, 480)
si.SetProperty2('ren1', 'resolution', 320, 240)
#si.SetProperty2('ren1', 'pixelsamples', 9, 9)
#Rendering
si.RenderScene('ren1')
#Output
si.SaveFrameBuffer('fb1',("../domefloor%03d" % f) + ".fb")
#Run commands
si.Run()
#si.Print()
1/2 >>