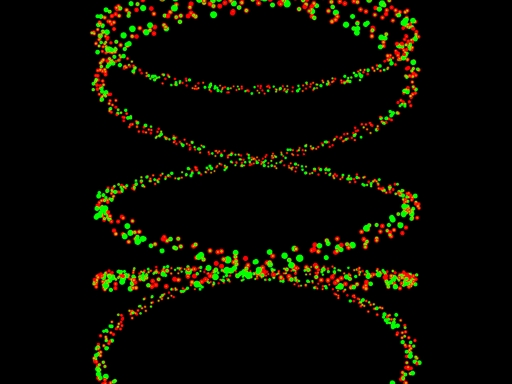
RenderMan Procedual Primitives
#width.py - Create Particles of different sizes
import random, math
import cgkit.cri
from cgkit.cgtypes import *
# Load the RenderMan API.
# Replace the library name with whatever renderer you want to use.
ri = cgkit.cri.loadRI("3delight")
cgkit.cri.importRINames(ri, globals())
COUNT = 2000
def jitter(scale):
val=random.random()*1000
return (val/500-1)*scale
position=[]
constantwidth=0.5
fov=30
red=[1,0,0]
green=[0,1,0]
width=[]
constantwidth=1.0
fov=30
#/*Generate Particle Postions*/
for i in range(COUNT):
x=math.sin(i*0.5)*50+jitter(2)
y=math.cos(i*0.1)*50+jitter(2)
z=math.cos(i*0.5)*100+jitter(2)
w=jitter(1.0)+0.5
position.append(vec3(x, y, z))
width.append(w)
RiBegin(RI_NULL)
RiDisplay ("width.tiff","file","rgb",RI_NULL)
RiFormat(512, 384, -1.0)
RiPixelSamples( 4, 4)
RiShadingRate(0.5)
RiProjection ("perspective","fov",fov,RI_NULL)
RiWorldBegin()
RiLightSource("ambientlight","intensity",0.4)
RiLightSource("distantlight", "from",[0,0,1])
RiTranslate(0,0,250)
RiSurface("plastic")
RiColor(red)
RiPoints("P",position,"constantwidth", constantwidth,RI_NULL)
RiColor(green)
RiPoints("P",position,"width",width,RI_NULL)
RiWorldEnd()
RiEnd()
- -
- -